树
在计算机科学中, 树(tree) 是一种广泛使用的抽象数据类型(ADT)— 或实现此 ADT 的数据结构 — 模拟分层树结构, 具有根节点和有父节点的子树,表示为一组链接节点。
树可以被(本地地)递归定义为一个(始于一个根节点的)节点集, 每个节点都是一个包含了值的数据结构, 除了值,还有该节点的节点引用列表(子节点)一起。
树的节点之间没有引用重复的约束。
一棵简单的无序树; 在下图中:
标记为 7 的节点具有两个子节点, 标记为 2 和 6;
一个父节点,标记为 2,作为根节点, 在顶部,没有父节点。
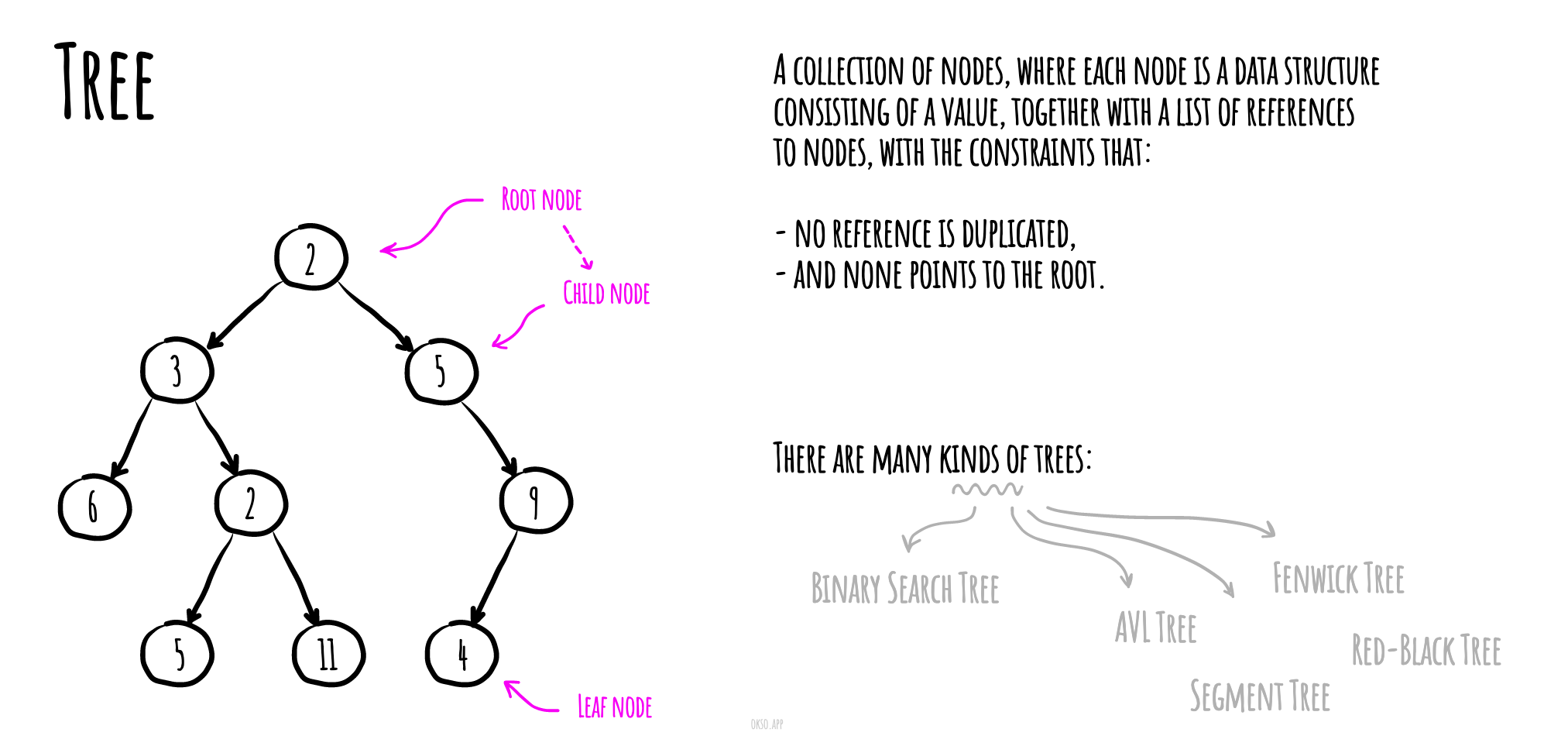
BinaryTreeNode
constructor
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
|
constructor(value = null) { this.left = null; this.right = null; this.parent = null; this.value = value;
this.meta = new HashTable();
this.nodeComparator = new Comparator(); }
|
leftHeight
1 2 3 4 5 6 7 8 9 10 11 12 13
|
get leftHeight() { if (!this.left) { return 0; }
return this.left.height + 1; }
|
rightHeight
1 2 3 4 5 6 7 8 9 10 11 12 13
|
get rightHeight() { if (!this.right) { return 0; }
return this.right.height + 1; }
|
height
1 2 3 4 5 6 7
|
get height() { return Math.max(this.leftHeight, this.rightHeight); }
|
balanceFactor
1 2 3 4 5 6 7
|
get balanceFactor() { return this.leftHeight - this.rightHeight; }
|
uncle
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
|
get uncle() { if (!this.parent) { return undefined; }
if (!this.parent.parent) { return undefined; }
if (!this.parent.parent.left || !this.parent.parent.right) { return undefined; }
if (this.nodeComparator.equal(this.parent, this.parent.parent.left)) { return this.parent.parent.right; }
return this.parent.parent.left; }
|
setValue(value)
1 2 3 4 5 6 7 8 9 10 11 12
|
setValue(value) { this.value = value;
return this; }
|
setLeft(node)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
|
setLeft(node) { if (this.left) { this.left.parent = null; }
this.left = node;
if (this.left) { this.left.parent = this; }
return this; }
|
setRight(node)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
|
setRight(node) { if (this.right) { this.right.parent = null; }
this.right = node;
if (node) { this.right.parent = this; }
return this; }
|
removeChild(nodeToRemove)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
|
removeChild(nodeToRemove) { if (this.left && this.nodeComparator.equal(this.left, nodeToRemove)) { this.left = null; return true; }
if (this.right && this.nodeComparator.equal(this.right, nodeToRemove)) { this.right = null; return true; }
return false; }
|
replaceChild(nodeToReplace, replacementNode)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
|
replaceChild(nodeToReplace, replacementNode) { if (!nodeToReplace || !replacementNode) { return false; }
if (this.left && this.nodeComparator.equal(this.left, nodeToReplace)) { this.left = replacementNode; return true; }
if (this.right && this.nodeComparator.equal(this.right, nodeToReplace)) { this.right = replacementNode; return true; }
return false; }
|
traverseInOrder
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
|
traverseInOrder() { let traverse = [];
if (this.left) { traverse = traverse.concat(this.left.traverseInOrder()); }
traverse.push(this.value);
if (this.right) { traverse = traverse.concat(this.right.traverseInOrder()); }
return traverse; }
|
toString
1 2 3 4 5 6
|
toString() { return this.traverseInOrder().toString(); }
|