红黑树
红黑树是一种自平衡的二叉搜索树,在计算机科学中使用。二叉树的每个节点都有一个额外的位,这个位通常被解释为节点的颜色(红色或黑色)。这些颜色位用于在插入和删除过程中保持树的大致平衡。
通过以一种满足特定属性的方式将树的每个节点标记为两种颜色之一,可以保持树的平衡,这些属性共同限制了树在最坏情况下可能变得不平衡的程度。当修改树时,新的树会被重新排列和重新标记以恢复颜色属性。这些属性的设计使得这种重新排列和重新标记可以高效地进行。
树的平衡并不完美,但足够好,可以确保在 O(log n)的时间内进行搜索,其中 n 是树中的元素总数。插入和删除操作以及树的重新排列和重新标记也可以在 O(log n)的时间内完成。
红黑树的一个示例:

属性
除了对二叉搜索树施加的要求之外,红黑树还必须满足以下条件:
- 每个节点要么是红色,要么是黑色。
- 根节点是黑色。这个规则有时可以省略。因为根节点总是可以从红色变为黑色,但不一定反之,所以这个规则对分析的影响很小。
- 所有叶子节点(NIL)都是黑色的。
- 如果一个节点是红色的,则它的两个子节点都是黑色的。
- 从给定节点到任何其后代 NIL 节点的每条路径都包含相同数量的黑色节点。
一些定义:从根节点到一个节点的黑色节点数称为该节点的黑色深度;从根节点到叶子节点的所有路径中的黑色节点数是红黑树的黑色高度。
这些约束条件强制实施了红黑树的一个关键特性:从根节点到最远叶子节点的路径长度不超过从根节点到最近叶子节点路径长度的两倍。结果是树大致上是高度平衡的。由于插入、删除和查找值等操作的最坏情况时间与树的高度成比例,这个对树高度的理论上限使得红黑树在最坏情况下具有高效性,而普通的二叉搜索树则不具备。
插入平衡
If uncle is RED

If uncle is BLACK
- 左左情况(p 是 g 的左子节点,x 是 p 的左子节点)
- 左右情况(p 是 g 的左子节点,x 是 p 的右子节点)
- 右右情况(p 是 g 的右子节点,x 是 p 的右子节点)
- 右左情况(p 是 g 的右子节点,x 是 p 的左子节点)
Left Left Case (See g, p and x)

Left Right Case (See g, p and x)

Right Right Case (See g, p and x)

Right Left Case (See g, p and x)
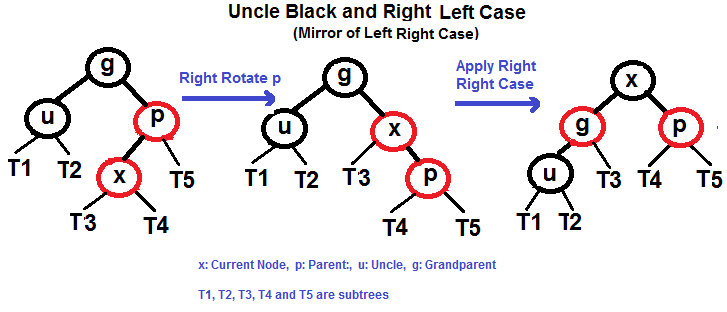
RedBlackTree
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317
| const RED_BLACK_TREE_COLORS = { red: "red", black: "black", };
const COLOR_PROP_NAME = "color";
export default class RedBlackTree extends BinarySearchTree {
insert(value) { const insertedNode = super.insert(value);
if (this.nodeComparator.equal(insertedNode, this.root)) { this.makeNodeBlack(insertedNode); } else { this.makeNodeRed(insertedNode); }
this.balance(insertedNode); return insertedNode; }
remove(value) { throw new Error(`Can't remove ${value}. Remove method is not implemented yet`); }
balance(node) { if (this.nodeComparator.equal(node, this.root)) { return; }
if (this.isNodeBlack(node.parent)) { return; }
const grandParent = node.parent.parent;
if (node.uncle && this.isNodeRed(node.uncle)) {
this.makeNodeBlack(node.uncle); this.makeNodeBlack(node.parent);
if (!this.nodeComparator.equal(grandParent, this.root)) { this.makeNodeRed(grandParent); } else { return; }
this.balance(grandParent); } else if (!node.uncle || this.isNodeBlack(node.uncle)) {
if (grandParent) { let newGrandParent;
if (this.nodeComparator.equal(grandParent.left, node.parent)) { if (this.nodeComparator.equal(node.parent.left, node)) { newGrandParent = this.leftLeftRotation(grandParent); } else { newGrandParent = this.leftRightRotation(grandParent); } } else { if (this.nodeComparator.equal(node.parent.right, node)) { newGrandParent = this.rightRightRotation(grandParent); } else { newGrandParent = this.rightLeftRotation(grandParent); } }
if (newGrandParent && newGrandParent.parent === null) { this.root = newGrandParent;
this.makeNodeBlack(this.root); }
this.balance(newGrandParent); } } }
leftLeftRotation(grandParentNode) { const grandGrandParent = grandParentNode.parent;
let grandParentNodeIsLeft; if (grandGrandParent) { grandParentNodeIsLeft = this.nodeComparator.equal(grandGrandParent.left, grandParentNode); }
const parentNode = grandParentNode.left;
const parentRightNode = parentNode.right;
parentNode.setRight(grandParentNode);
grandParentNode.setLeft(parentRightNode);
if (grandGrandParent) { if (grandParentNodeIsLeft) { grandGrandParent.setLeft(parentNode); } else { grandGrandParent.setRight(parentNode); } } else { parentNode.parent = null; }
this.swapNodeColors(parentNode, grandParentNode);
return parentNode; }
leftRightRotation(grandParentNode) { const parentNode = grandParentNode.left; const childNode = parentNode.right;
const childLeftNode = childNode.left;
childNode.setLeft(parentNode);
parentNode.setRight(childLeftNode);
grandParentNode.setLeft(childNode);
return this.leftLeftRotation(grandParentNode); }
rightRightRotation(grandParentNode) { const grandGrandParent = grandParentNode.parent;
let grandParentNodeIsLeft; if (grandGrandParent) { grandParentNodeIsLeft = this.nodeComparator.equal(grandGrandParent.left, grandParentNode); }
const parentNode = grandParentNode.right;
const parentLeftNode = parentNode.left;
parentNode.setLeft(grandParentNode);
grandParentNode.setRight(parentLeftNode);
if (grandGrandParent) { if (grandParentNodeIsLeft) { grandGrandParent.setLeft(parentNode); } else { grandGrandParent.setRight(parentNode); } } else { parentNode.parent = null; }
this.swapNodeColors(parentNode, grandParentNode);
return parentNode; }
rightLeftRotation(grandParentNode) { const parentNode = grandParentNode.right; const childNode = parentNode.left;
const childRightNode = childNode.right;
childNode.setRight(parentNode);
parentNode.setLeft(childRightNode);
grandParentNode.setRight(childNode);
return this.rightRightRotation(grandParentNode); }
makeNodeRed(node) { node.meta.set(COLOR_PROP_NAME, RED_BLACK_TREE_COLORS.red);
return node; }
makeNodeBlack(node) { node.meta.set(COLOR_PROP_NAME, RED_BLACK_TREE_COLORS.black);
return node; }
isNodeRed(node) { return node.meta.get(COLOR_PROP_NAME) === RED_BLACK_TREE_COLORS.red; }
isNodeBlack(node) { return node.meta.get(COLOR_PROP_NAME) === RED_BLACK_TREE_COLORS.black; }
isNodeColored(node) { return this.isNodeRed(node) || this.isNodeBlack(node); }
swapNodeColors(firstNode, secondNode) { const firstColor = firstNode.meta.get(COLOR_PROP_NAME); const secondColor = secondNode.meta.get(COLOR_PROP_NAME);
firstNode.meta.set(COLOR_PROP_NAME, secondColor); secondNode.meta.set(COLOR_PROP_NAME, firstColor); } }
|