堆 (数据结构)
在计算机科学中, 一个 堆(heap) 是一种特殊的基于树的数据结构,它满足下面描述的堆属性。
在一个 最小堆(min heap) 中, 如果 P
是 C
的一个父级节点, 那么 P
的 key(或 value)应小于或等于 C
的对应值.
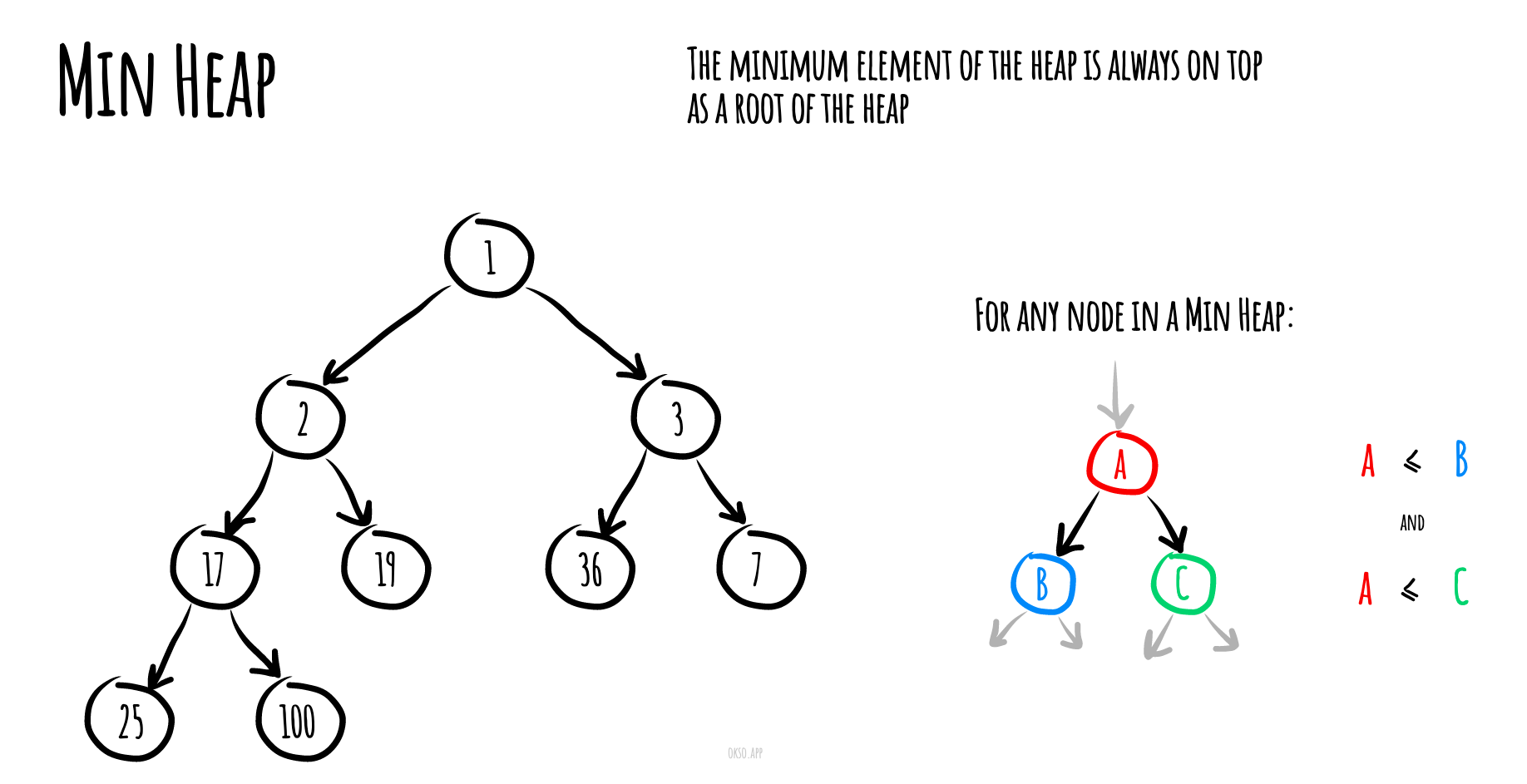
在一个 最大堆(max heap) 中, P
的 key(或 value)大于 C
的对应值。
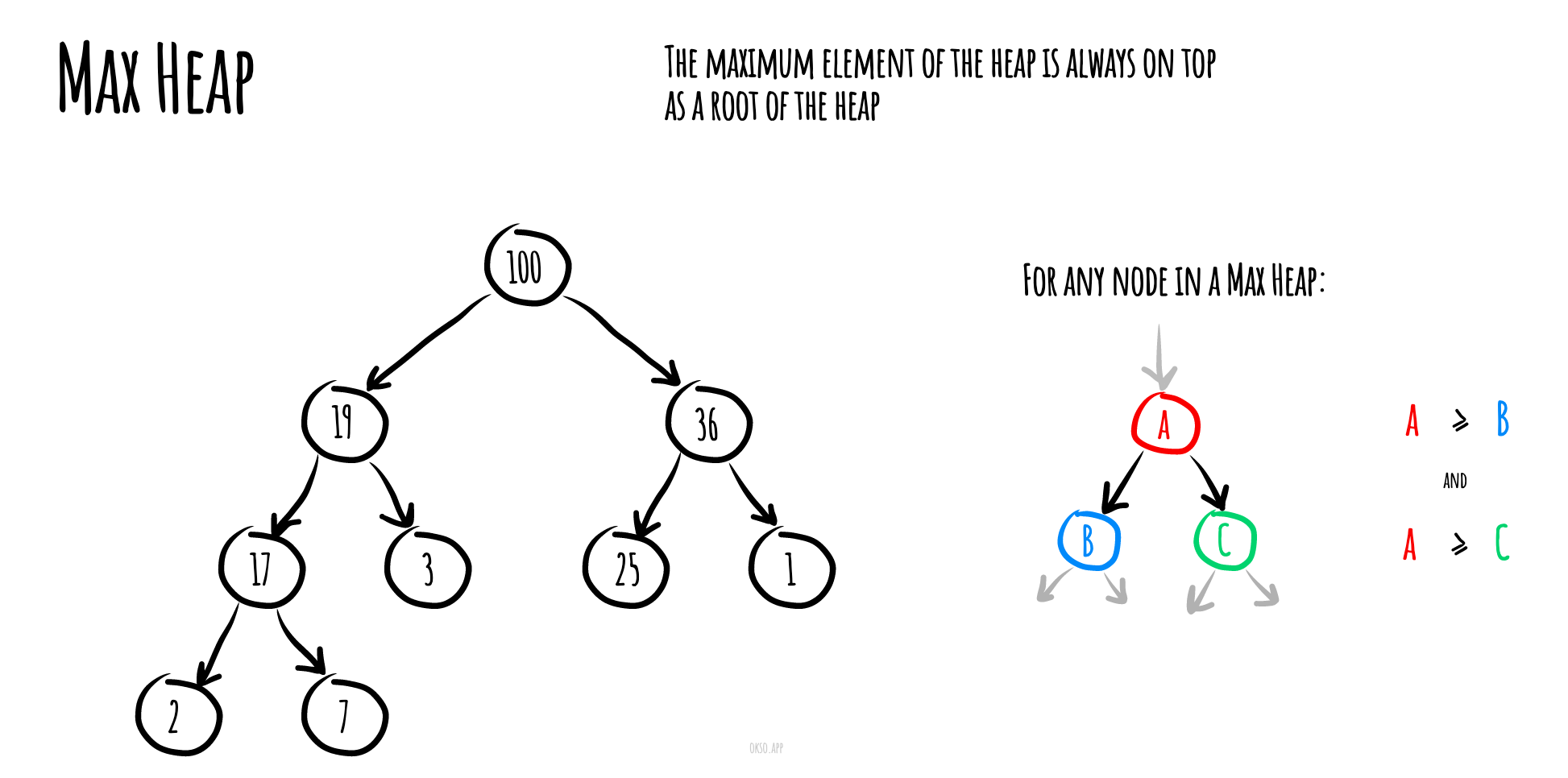
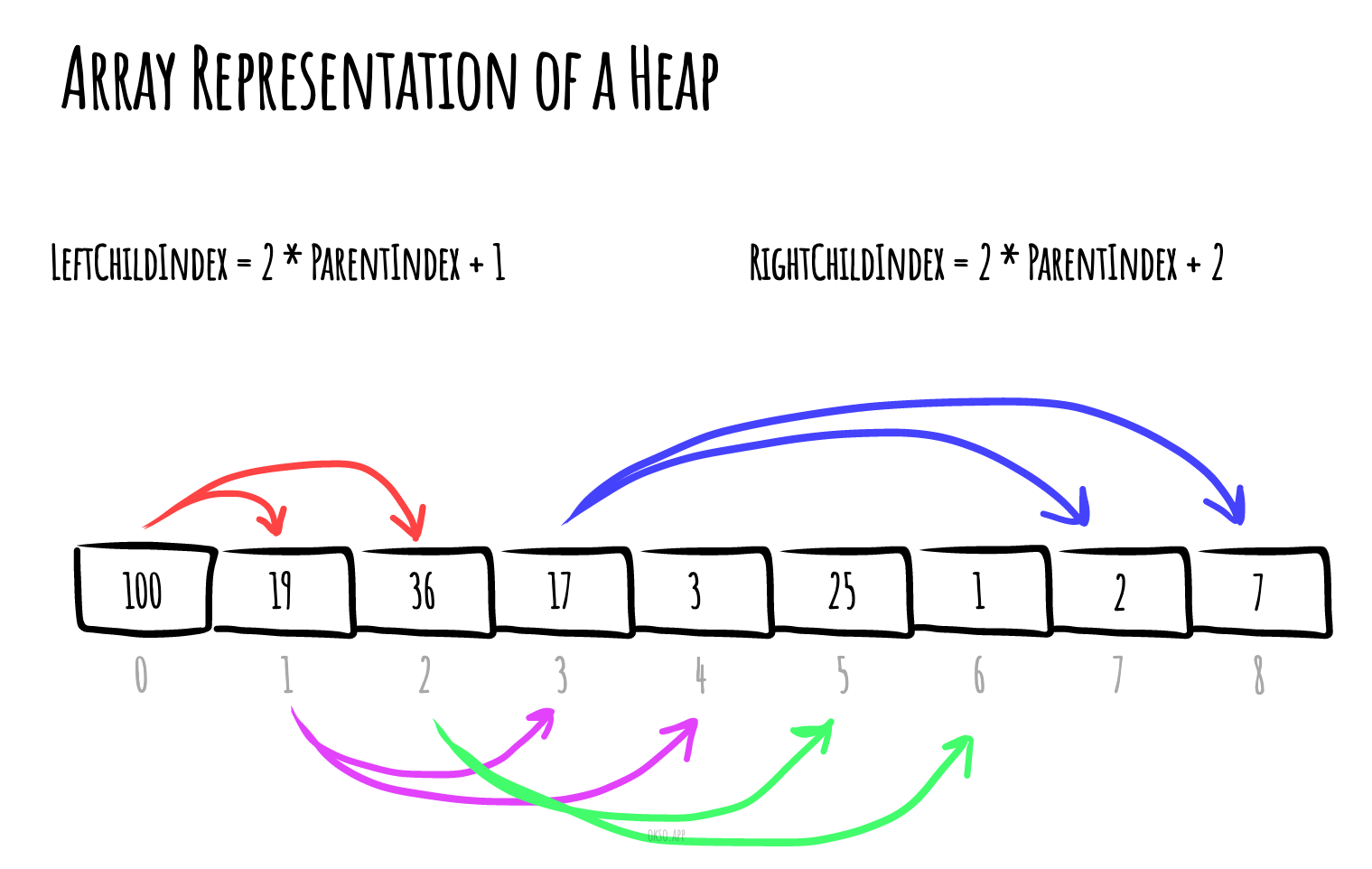
在堆“顶部”的没有父级节点的节点,被称之为根节点。
Heap 类
构造函数 constructor
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
|
constructor(comparatorFunction) { if (new.target === Heap) { throw new TypeError('Cannot construct Heap instance directly'); }
this.heapContainer = [];
this.compare = new Comparator(comparatorFunction); }
|
getLeftChildIndex(parentIndex) 方法
1 2 3 4 5 6 7
|
getLeftChildIndex(parentIndex) { return (2 * parentIndex) + 1; }
|
getRightChildIndex(parentIndex) 方法
1 2 3 4 5 6 7
|
getRightChildIndex(parentIndex) { return (2 * parentIndex) + 2; }
|
getParentIndex(childIndex) 方法
1 2 3 4 5 6 7
|
getParentIndex(childIndex) { return Math.floor((childIndex - 1) / 2); }
|
hasParent(childIndex) 方法
1 2 3 4 5 6 7 8 9
|
hasParent(childIndex) { return this.getParentIndex(childIndex) >= 0; }
|
hasLeftChild(parentIndex) 方法
1 2 3 4 5 6 7 8
|
hasLeftChild(parentIndex) { return this.getLeftChildIndex(parentIndex) < this.heapContainer.length; }
|
hasRightChild(parentIndex) 方法
1 2 3 4 5 6 7 8
|
hasRightChild(parentIndex) { return this.getRightChildIndex(parentIndex) < this.heapContainer.length; }
|
leftChild(parentIndex) 方法
1 2 3 4 5 6 7
|
leftChild(parentIndex) { return this.heapContainer[this.getLeftChildIndex(parentIndex)]; }
|
rightChild(parentIndex) 方法
1 2 3 4 5 6 7 8 9
|
rightChild(parentIndex) { return this.heapContainer[this.getRightChildIndex(parentIndex)]; }
|
parent(childIndex) 方法
1 2 3 4 5 6 7 8 9 10
|
parent(childIndex) { const parentIndex = this.getParentIndex(childIndex); return this.heapContainer[parentIndex]; }
|
swap(indexOne, indexTwo) 方法
1 2 3 4 5 6 7 8 9
|
swap(indexOne, indexTwo) { const tmp = this.heapContainer[indexTwo]; this.heapContainer[indexTwo] = this.heapContainer[indexOne]; this.heapContainer[indexOne] = tmp; }
|
peek() 方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14
|
peek() { if (this.heapContainer.length === 0) { return null; }
return this.heapContainer[0]; }
|
poll() 方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
|
poll() { if (this.heapContainer.length === 0) { return null; }
if (this.heapContainer.length === 1) { return this.heapContainer.pop(); }
const item = this.heapContainer[0];
this.heapContainer[0] = this.heapContainer.pop();
this.heapifyDown();
return item; }
|
add(item) 方法
1 2 3 4 5 6 7 8 9 10
|
add(item) { this.heapContainer.push(item); this.heapifyUp(); return this; }
|
remove(item, comparator = this.compare) 方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
|
remove(item, comparator = this.compare) { const numberOfItemsToRemove = this.find(item, comparator).length;
for (let iteration = 0; iteration < numberOfItemsToRemove; iteration += 1) { const indexToRemove = this.find(item, comparator).pop();
if (indexToRemove === (this.heapContainer.length - 1)) { this.heapContainer.pop(); } else { this.heapContainer[indexToRemove] = this.heapContainer.pop();
const parentItem = this.parent(indexToRemove);
if ( this.hasLeftChild(indexToRemove) && ( !parentItem || this.pairIsInCorrectOrder(parentItem, this.heapContainer[indexToRemove]) ) ) { this.heapifyDown(indexToRemove); } else { this.heapifyUp(indexToRemove); } } }
return this; }
|
find(item, comparator = this.compare) 方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
|
find(item, comparator = this.compare) { const foundItemIndices = [];
for (let itemIndex = 0; itemIndex < this.heapContainer.length; itemIndex += 1) { if (comparator.equal(item, this.heapContainer[itemIndex])) { foundItemIndices.push(itemIndex); } }
return foundItemIndices; }
|
isEmpty() 方法
1 2 3 4 5 6 7
|
isEmpty() { return !this.heapContainer.length; }
|
toString() 方法
1 2 3 4 5 6 7
|
toString() { return this.heapContainer.toString(); }
|
heapifyUp(customStartIndex) 方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
|
heapifyUp(customStartIndex) { let currentIndex = customStartIndex || this.heapContainer.length - 1;
while ( this.hasParent(currentIndex) && !this.pairIsInCorrectOrder(this.parent(currentIndex), this.heapContainer[currentIndex]) ) { this.swap(currentIndex, this.getParentIndex(currentIndex)); currentIndex = this.getParentIndex(currentIndex); } }
|
该方法使用一个 while 循环来比较当前元素与其父元素,并在它们不满足正确的顺序关系时进行交换。循环会一直执行,直到当前元素到达正确的位置或成为堆的根节点。
heapifyDown(customStartIndex = 0) 方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
|
heapifyDown(customStartIndex = 0) { let currentIndex = customStartIndex; let nextIndex = null;
while (this.hasLeftChild(currentIndex)) { if ( this.hasRightChild(currentIndex) && this.pairIsInCorrectOrder(this.rightChild(currentIndex), this.leftChild(currentIndex)) ) { nextIndex = this.getRightChildIndex(currentIndex); } else { nextIndex = this.getLeftChildIndex(currentIndex); }
if (this.pairIsInCorrectOrder( this.heapContainer[currentIndex], this.heapContainer[nextIndex], )) { break; }
this.swap(currentIndex, nextIndex); currentIndex = nextIndex; } }
|
这个方法是用于执行堆向下调整的过程。它接受一个可选的参数 customStartIndex
,用于指定开始堆化的索引位置,默认为 0。该方法比较父节点与其子节点,并根据堆的类型(最小堆或最大堆)与合适的子节点交换位置。然后,它继续对交换后的子节点进行相同的操作,直到元素在堆中达到正确的位置为止。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
|
pairIsInCorrectOrder(firstElement, secondElement) { throw new Error(` You have to implement heap pair comparision method for ${firstElement} and ${secondElement} values. `); }
|
这段代码用于检查堆中的元素对是否按正确的顺序排列。根据堆的类型,有两种情况:
对于 MinHeap
,第一个元素必须始终小于或等于第二个元素。
对于 MaxHeap
,第一个元素必须始终大于或等于第二个元素。
该函数接受两个参数,表示需要进行比较的元素对。然后,它抛出一个错误,并提供了一个错误消息,指示需要实现堆元素对比较的方法。错误消息中包含了第一个元素和第二个元素的值。
MaxHeap 类
pairIsInCorrectOrder(firstElement, secondElement) 方法
1 2 3 4 5 6 7 8 9 10 11 12
|
pairIsInCorrectOrder(firstElement, secondElement) { return this.compare.greaterThanOrEqual(firstElement, secondElement); }
|
这个方法用于检查堆元素对是否按照正确的顺序排列。根据堆的类型(最小堆或最大堆),第一个元素必须始终小于或等于第二个元素(最小堆),或者第一个元素必须始终大于或等于第二个元素(最大堆)。
MinHeap 类
pairIsInCorrectOrder(firstElement, secondElement) 方法
1 2 3 4 5 6 7 8 9 10 11 12 13
|
pairIsInCorrectOrder(firstElement, secondElement) { return this.compare.lessThanOrEqual(firstElement, secondElement); }
|